Thursday 3 May 2012
Thursday 23 February 2012
JDBC - ODBC Connection Example - JDBC Basics – Java Database Connectivity Steps | Java JDBC Connection Example - JDBC Driver Example
The JDBC ( Java Database Connectivity) API defines interfaces and
classes for writing database applications in Java by making database
connections. Using JDBC you can send SQL, PL/SQL statements to almost
any relational database. JDBC is a Java API for executing SQL statements
and supports basic SQL functionality. It provides RDBMS access by
allowing you to embed SQL inside Java code. Because Java can run on a
thin client, applets embedded in Web pages can contain downloadable JDBC
code to enable remote database access. You will learn how to create a
table, insert values into it, query the table, retrieve results, and
update the table with the help of a JDBC Program example.
java.sql package.
import java.sql.*; The star ( * ) indicates that all of the classes in the package java.sql are to be imported.
The return type of the Class.forName (String ClassName) method is “Class”. Class is a class in
java.lang package.
2. Creating a oracle jdbc Connection
The JDBC DriverManager class defines objects which can connect Java applications to a JDBC driver. DriverManager is considered the backbone of JDBC architecture. DriverManager class manages the JDBC drivers that are installed on the system. Its getConnection() method is used to establish a connection to a database. It uses a username, password, and a jdbc url to establish a connection to the database and returns a connection object. A jdbc Connection represents a session/connection with a specific database. Within the context of a Connection, SQL, PL/SQL statements are executed and results are returned. An application can have one or more connections with a single database, or it can have many connections with different databases. A Connection object provides metadata i.e. information about the database, tables, and fields. It also contains methods to deal with transactions.
JDBC URL Example:: jdbc: <subprotocol>: <subname>•Each driver has its own subprotocol
•Each subprotocol has its own syntax for the source. We’re using the jdbc odbc subprotocol, so the DriverManager knows to use the sun.jdbc.odbc.JdbcOdbcDriver.
Once a connection is obtained we can interact with the database. Connection interface defines methods for interacting with the database via the established connection. To execute SQL statements, you need to instantiate a Statement object from your connection object by using the createStatement() method.
Statement statement = dbConnection.createStatement();
A statement object is used to send and execute SQL statements to a database.
Statement: Execute simple sql queries without parameters.
Statement createStatement()
Creates an SQL Statement object.
Prepared Statement: Execute precompiled sql queries with or without parameters.
PreparedStatement prepareStatement(String sql)
returns a new PreparedStatement object. PreparedStatement objects are precompiled
SQL statements.
Callable Statement: Execute a call to a database stored procedure.
CallableStatement prepareCall(String sql)
returns a new CallableStatement object. CallableStatement objects are SQL stored procedure call statements.
executeQuery(), executeUpdate(), and execute(). For a SELECT statement, the method to use is executeQuery . For statements that create or modify tables, the method to use is executeUpdate. Note: Statements that create a table, alter a table, or drop a table are all examples of DDL
statements and are executed with the method executeUpdate. execute() executes an SQL
statement that is written as String object.
ResultSet provides access to a table of data generated by executing a Statement. The table rows are retrieved in sequence. A ResultSet maintains a cursor pointing to its current row of data. The next() method is used to successively step through the rows of the tabular results.
ResultSetMetaData Interface holds information on the types and properties of the columns in a ResultSet. It is constructed from the Connection object.
JDBC Example :
JDBC Architecture
JDBC Basics – Java Database Connectivity Steps
Before you can create a java jdbc connection to the database, you must first import thejava.sql package.
import java.sql.*; The star ( * ) indicates that all of the classes in the package java.sql are to be imported.
1. Loading a database driver,
In this step of the jdbc connection process, we load the driver class
by calling Class.forName() with the Driver class name as an argument.
Once loaded, the Driver class creates an instance of itself. A client
can connect to Database Server through JDBC Driver. Since most of the
Database servers support ODBC driver therefore JDBC-ODBC Bridge driver
is commonly used.The return type of the Class.forName (String ClassName) method is “Class”. Class is a class in
java.lang package.
try {
Class.forName(”sun.jdbc.odbc.JdbcOdbcDriver”); //Or any other driver
}
catch(Exception x){
System.out.println( “Unable to load the driver class!” );
}
|
2. Creating a oracle jdbc Connection
The JDBC DriverManager class defines objects which can connect Java applications to a JDBC driver. DriverManager is considered the backbone of JDBC architecture. DriverManager class manages the JDBC drivers that are installed on the system. Its getConnection() method is used to establish a connection to a database. It uses a username, password, and a jdbc url to establish a connection to the database and returns a connection object. A jdbc Connection represents a session/connection with a specific database. Within the context of a Connection, SQL, PL/SQL statements are executed and results are returned. An application can have one or more connections with a single database, or it can have many connections with different databases. A Connection object provides metadata i.e. information about the database, tables, and fields. It also contains methods to deal with transactions.
JDBC URL Syntax:: jdbc: <subprotocol>: <subname>
|
JDBC URL Example:: jdbc: <subprotocol>: <subname>•Each driver has its own subprotocol
•Each subprotocol has its own syntax for the source. We’re using the jdbc odbc subprotocol, so the DriverManager knows to use the sun.jdbc.odbc.JdbcOdbcDriver.
try{
Connection dbConnection=DriverManager.getConnection(url,”loginName”,”Password”)
}
catch( SQLException x ){
System.out.println( “Couldn’t get connection!” );
}
3. Creating a jdbc Statement object,
Once a connection is obtained we can interact with the database. Connection interface defines methods for interacting with the database via the established connection. To execute SQL statements, you need to instantiate a Statement object from your connection object by using the createStatement() method.
Statement statement = dbConnection.createStatement();
A statement object is used to send and execute SQL statements to a database.
Three kinds of Statements
Statement createStatement()
Creates an SQL Statement object.
Prepared Statement: Execute precompiled sql queries with or without parameters.
PreparedStatement prepareStatement(String sql)
returns a new PreparedStatement object. PreparedStatement objects are precompiled
SQL statements.
Callable Statement: Execute a call to a database stored procedure.
CallableStatement prepareCall(String sql)
returns a new CallableStatement object. CallableStatement objects are SQL stored procedure call statements.
4. Executing a SQL statement with the Statement object, and returning a jdbc resultSet.
Statement interface defines methods that are used to interact with
database via the execution of SQL statements. The Statement class has
three methods for executing statements:executeQuery(), executeUpdate(), and execute(). For a SELECT statement, the method to use is executeQuery . For statements that create or modify tables, the method to use is executeUpdate. Note: Statements that create a table, alter a table, or drop a table are all examples of DDL
statements and are executed with the method executeUpdate. execute() executes an SQL
statement that is written as String object.
ResultSet provides access to a table of data generated by executing a Statement. The table rows are retrieved in sequence. A ResultSet maintains a cursor pointing to its current row of data. The next() method is used to successively step through the rows of the tabular results.
ResultSetMetaData Interface holds information on the types and properties of the columns in a ResultSet. It is constructed from the Connection object.
Test JDBC Driver Installation
import javax.swing.JOptionPane;
public class TestJDBCDriverInstallation_Oracle {
public static void main(String[] args) {
StringBuffer output = new StringBuffer();
output.append(”Testing oracle driver installation \n”);
try {
String className = “sun.jdbc.odbc.JdbcOdbcDriver”;
Class driverObject = Class.forName(className);
output.append(”Driver : “+driverObject+”\n”);
output.append(”Driver Installation Successful”);
JOptionPane.showMessageDialog(null, output);
} catch (Exception e) {
output = new StringBuffer();
output.append(”Driver Installation FAILED\n”);
JOptionPane.showMessageDialog(null, output);
System.out.println(”Failed: Driver Error: ” + e.getMessage());
}
}
}
JDBC Example :
import java.sql.* ; class JDBCQuery { public static void main( String args[] ) { try { // Load the database driver Class.forName( "sun.jdbc.odbc.JdbcOdbcDriver" ) ; // Get a connection to the database Connection conn = DriverManager.getConnection( "jdbc:odbc:Database" ) ; // Print all warnings for( SQLWarning warn = conn.getWarnings(); warn != null; warn = warn.getNextWarning() ) { System.out.println( "SQL Warning:" ) ; System.out.println( "State : " + warn.getSQLState() ) ; System.out.println( "Message: " + warn.getMessage() ) ; System.out.println( "Error : " + warn.getErrorCode() ) ; } // Get a statement from the connection Statement stmt = conn.createStatement() ; // Execute the query ResultSet rs = stmt.executeQuery( "SELECT * FROM Cust" ) ; // Loop through the result set while( rs.next() ) System.out.println( rs.getString(1) ) ; // Close the result set, statement and the connection rs.close() ; stmt.close() ; conn.close() ; } catch( SQLException se ) { System.out.println( "SQL Exception:" ) ; // Loop through the SQL Exceptions while( se != null ) { System.out.println( "State : " + se.getSQLState() ) ; System.out.println( "Message: " + se.getMessage() ) ; System.out.println( "Error : " + se.getErrorCode() ) ; se = se.getNextException() ; } } catch( Exception e ) { System.out.println( e ) ; } } }
Wednesday 22 February 2012
What is Microsoft .Net Framework | What is .Net ?
The Microsoft .Net Framework is a platform that
provides tools and technologies you need to build Networked Applications
as well as Distributed Web Services and Web Applications. The .Net
Framework provides the necessary compile time and run-time foundation to
build and run any language that conforms to the Common Language Specification (CLS).The main two components of .Net Framework are Common Language Runtime (CLR) and .Net Framework Class Library (FCL).
The Common Language Runtime
(CLR) is the runtime environment of the .Net Framework , that executes
and manages all running code like a Virtual Machine. The .Net Framework Class Library
(FCL) is a huge collection of language-independent and type-safe
reusable classes. The .Net Framework Class Libraries (FCL) are arranged
into a logical grouping according to their functionality and usability
is called Namespaces. In the following sections describes how to .Net Framework manages the code in compile time and run time .
Microsoft .NET (pronounced “dot net”) is a software component that
runs on the Windows operating system. .NET provides tools and libraries
that enable developers to create Windows software much faster and
easier. .NET benefits end-users by providing applications of higher
capability, quality and security. The .NET Framework must be installed
on a user’s PC to run .NET applications.
This is how Microsoft describes it: “.NET is the Microsoft Web
services strategy to connect information, people, systems, and devices
through software. Integrated across the Microsoft platform, .NET
technology provides the ability to quickly build, deploy, manage, and
use connected, security-enhanced solutions with Web services.
.NET-connected solutions enable businesses to integrate their systems
more rapidly and in a more agile manner and help them realize the
promise of information anytime, anywhere, on any device.” See Microsoft for more information.
.NET is both a business strategy from Microsoft and its collection of programming support for what are known as Web services,
the ability to use the Web rather than your own computer for various
services. Microsoft's goal is to provide individual and business users
with a seamlessly interoperable and Web-enabled interface for
applications and computing devices and to make computing activities
increasingly Web browser-oriented.
The .NET platform includes servers; building-block services, such as
Web-based data storage; and device software. It also includes Passport,
Microsoft's fill-in-the-form-only-once identity verification service.
- The ability to make the entire range of computing devices work together and to have user information automatically updated and synchronized on all of them
- Increased interactive capability for Web sites, enabled by greater use of XML (Extensible Markup Language) rather than HTML
- A premium online subscription service, that will feature customized access and delivery of products and services to the user from a central starting point for the management of various applications, such as e-mail, for example, or software, such as Office .NET
- Centralized data storage, which will increase efficiency and ease of access to information, as well as synchronization of information among users and devices
- The ability to integrate various communications media, such as e-mail, faxes, and telephones
- For developers, the ability to create reusable modules, which should increase productivity and reduce the number of programming errors
The full release of .NET is expected to take several years to complete, with intermittent releases of products such as a personal security service and new versions of Windows and Office that implement the .NET strategy coming on the market separately. Visual Studio .NET is a development environment that is now available. Windows XP supports certain .NET capabilities.
What Is PHP ? | Example of php - Hyper Text Preprocessor
PHP (recursive acronym for PHP: Hypertext
Preprocessor) is a widely-used open source general-purpose
scripting language that is especially suited for web
development and can be embedded into HTML.
- PHP stands for PHP: Hypertext Preprocessor
- PHP is a server-side scripting language, like ASP
- PHP scripts are executed on the server
- PHP supports many databases (MySQL, Informix, Oracle, Sybase, Solid, PostgreSQL, Generic ODBC, etc.)
- PHP is an open source software
- PHP is free to download and use
PHP is a server-side scripting language
for creating dynamic Web pages. You create pages with PHP and HTML. When
a visitor opens the page, the server processes the PHP commands and
then sends the results to the visitor's browser, just as with ASP or
ColdFusion. Unlike ASP or ColdFusion, however, PHP is Open Source and
cross-platform. PHP runs on Windows NT and many Unix versions, and it
can be built as an Apache module and as a binary that can run as a CGI.
When built as an Apache module, PHP is especially lightweight and
speedy. Without any process creation overhead, it can return results
quickly, but it doesn't require the tuning of mod_perl to keep your server's memory image small.
In addition to manipulating the content of your pages, PHP can also send HTTP headers. You can set cookies, manage authentication, and redirect users. It offers excellent connectivity to many databases (and ODBC), and integration with various external libraries that let you do everything from generating PDF documents to parsing XML.
PHP goes right into your Web pages, so there's no need for a special development environment or IDE. You start a block of PHP code with <?php and end it with ?>. (You can also configure PHP to use ASP-style <% %> tags or even <SCRIPT LANGUAGE="php"></SCRIPT>.) The PHP engine processes everything between those tags.
PHP's language syntax is similar to C's and Perl's. You don't have to declare variables before you use them, and it's easy to create arrays and hashes (associative arrays). PHP even has some rudimentary object-oriented features, providing a helpful way to organize and encapsulate your code.
Although PHP runs fastest embedded in Apache, there are instructions on the PHP Web site for seamless setup with Microsoft IIS and Netscape Enterprise Server. If you don't already have a copy of PHP, you can download it at the official Web site. You'll also find a manual that documents all of PHP's functions and features.
Example :
In addition to manipulating the content of your pages, PHP can also send HTTP headers. You can set cookies, manage authentication, and redirect users. It offers excellent connectivity to many databases (and ODBC), and integration with various external libraries that let you do everything from generating PDF documents to parsing XML.
PHP goes right into your Web pages, so there's no need for a special development environment or IDE. You start a block of PHP code with <?php and end it with ?>. (You can also configure PHP to use ASP-style <% %> tags or even <SCRIPT LANGUAGE="php"></SCRIPT>.) The PHP engine processes everything between those tags.
PHP's language syntax is similar to C's and Perl's. You don't have to declare variables before you use them, and it's easy to create arrays and hashes (associative arrays). PHP even has some rudimentary object-oriented features, providing a helpful way to organize and encapsulate your code.
Although PHP runs fastest embedded in Apache, there are instructions on the PHP Web site for seamless setup with Microsoft IIS and Netscape Enterprise Server. If you don't already have a copy of PHP, you can download it at the official Web site. You'll also find a manual that documents all of PHP's functions and features.
Example :
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN"
"http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<title>Example</title>
</head>
<body>
<?php
echo "Hi, I'm a PHP script!";
?>
</body>
</html>
Monday 13 February 2012
Mac - Window 7 Desktop - HD Wallpapers Free Download - Mac - Window 7 Desktop Themes - HD Wallpapers - VAP WALLPAPERS
Open Spot - Google's New Android Application - VAP'S Internet Information
Breaking News! Google released a new Android application called 'Open
Spot' for the users to find and share parking. By the help the new app,
the users will be able to update the current map regarding the parking
spots.
The new app 'Open Spot' is quite simple. It displays open parking spots within a 1.5km/0.9 mile radius of where you are. The spots are coded with colour and will be displayed for twenty minutes only according to users' time setting.
However, there are some limitations in the application. The spot will appear marked as 'fresh' within five minutes. After that, there may not be availability of the spot for parking. On the other hand, the application will only be useful if it is adopted on large scale.
The new app 'Open Spot' is quite simple. It displays open parking spots within a 1.5km/0.9 mile radius of where you are. The spots are coded with colour and will be displayed for twenty minutes only according to users' time setting.
However, there are some limitations in the application. The spot will appear marked as 'fresh' within five minutes. After that, there may not be availability of the spot for parking. On the other hand, the application will only be useful if it is adopted on large scale.
Diffiernce between Google.com and Google.co.in - VAP'S INFORMATION
Diffiernce between Google.com and Google.co.in
Most
of you might have felt this difference between Google's Indian and
Worldwide Sites. When ever you openGoogle in India, automatically
google.co.in opens - The Indian Version of Google. In the US of A
google.com opens up giving a totally different search experience and
algorithms. Google algorithms for Indians are totally aligned to the
Desi Culture and their Foxy Habits ;)
Now we researched on a typical foxy word REMOVING on Google's Indian and International Versions. Below are the Astonishing results ;)
This first snapshot if from GOOGLE USA where the work REMOVING suggests a totally different aspect of culture in America.
Now below is what REMOVING rhymes to the Indians ;)
Send Free Valentine SMS - Valentine's Day SMS in Hindi - English - Greetings. | Love Sms Quota - Valentine Special SMS - VISHAL PANDYA
Valentine's Day SMS in Hindi
1) Har Subah apki FINE hoDildar dosto ki LINE ho
Zindagi me khushi ki SIGN ho
Bhagwan kare aapka har din VALENTINE ho
2) Lamha Lamha waqt guzer jayega
3 Din bad Valentine Day aa jayega
Abhi B waqt he kisi se affair chala lo
Warana ye Valentine’s Day B Bekar Chala Jaega
3) Pyar shabdo ka mohtaj nhi hota
Dil me har kisi ka raj nahi hota
Kyu intezar karte he sabhi Valentine’s Day ka
Kya sal ka har din pyar ka haqdar ni hota?
4) Yaar Mujhe rk baat batao
14 Feb. ko Valentine's Day manane ke theek
9 mahine baad 14 November Ko
"Baal-Divas" kyo aata hai
5) Ladki- ish 'Valentine’s Day' pe mujhe
Aisa gift chahiye jo sab se mehnga hai
Ladka- thik hai, Dunga
Ladki- Kya doge
Ladka- sharmate huye
'PYAAZ'
6) Boy: aisa valentine cards milega jisme likha ho
"Tum hi mera pehala Pyar ho"
Dukandar: Yes
Ladka- Thik he 10 card de do
Valentine's Day SMS in English
1) My Valentine, you're all I wantIn you, I find joy and delight
You give me everything I need
I'm happiest when you're in sight.
2) The Best Valentine’s Day gift this YEAR is to gift ONIONS
Because,
A. Get color of Love (Pink)
B. High Market Value
V. You can see tears of happiness
3) V - Very
A - Adorable
L - Lovely
E - Exotic
N - Nice
T - Ticklish
I - Interesting
N - Naughty
E - Exceptional Day
Happy Valentine’s Day 2011 in advance
I though to bring lot of flowers and happyness for this valentines day but you said i am not your dear not even a friend to wish you...
Happy valentines day dear..
-----------------------
Jaise Hawa Ka Jhonka, Shehad Ki Mithaas, Jaise Phoolon Ki Khushbu,Jaise Pyar, Jante Ho Sub Se Khubsurat Ehsas Kya Hai, Aap Ka Sath…..! I Love You Happy Valentines Day
-----------------------
My rose is red,
Ur eyes r blue,
You love me,
and I love u.
Happy valentine’s day.
-----------------------
Anyone can catch your eye, but it takes someone special to catch your heart. Happy Valentine's Day
-----------------------
Some People are So Much Important in our Life.
Not because We Enjoy their Company.
But Because We Feel Sooo much Loneliness in their Absence.
Happy Valentine's Day.
-----------------------
This day of love I take a vow
To love you well through all the days
Of long and labyrinthine ways:
But I would love you anyhow
Because You r
My Valentine.
-----------------------
Love comes
through the hands
that touch
With
unabashed affection,
For only
skin-to-skin can love
Maintain
its true direction.
I love u my Valentine.
-----------------------
This is not a special day for me because i celebrate my every day like a "Velentine Day" since you are with me............
Happy Valentine Day......
-----------------------
In life there r 5 things u should never lose
Character
Self respect
Hope
Heart
.
.
.
&
.
well U know my name
So Be My Valentine.
-----------------------
May the Valentine's Day spirit fill your heart
With love and joy on this special day.
A time to exchange messages - a spirit of love,
Sentimental verses sent in a poetic way.
-----------------------
If you would be my valentine
My heart would surely glow
And if you'd be my valentine
I'd want the world to know.
-----------------------
Valentine Oh Valentine…..,
Darlin’ won’t you please be mine….,
My love for you is on the line…..,
Lovely Valentine….!
-----------------------
On this day made just for lovers
something weighs heavily on my mind
yet still I have to question
.
.
.
Will you be my valentine?
-----------------------
I wish to live in ur eyes
Die in ur arms
&
Be buried in ur heart
Happy Valentine's day
-----------------------
You are unique
You are caring and
You are the best. And I am the luckiest to have you in my life!
Happy Valentine's Day my sweet heart!
-----------------------
I'm sending u a Valentine's Wish
Filled with Hugs and Kisses too.
Because there is a special place
Within my heart for u.
-----------------------
Oh! the happy bounding flea
You cannot tell the he from she
But she can tell
And so can he
Whoopee !
From one bed bug to another Happy Valentine's Day
-----------------------
Love is like playing the piano.
First you must learn to
play by the rules,
then you must forget the
rules and play from your heart.
Happy Valentine's Day
-----------------------
I love my life
Because it gave me you
I love you
Because you are my life
Happy Valentine's Day
-----------------------
HAPPY VALENTINES DAY BUT TODAY NOT ONLY GREET UR LUV ONES BUT ALSO TELL THEM HOW MUCH U LUV THEM!!!!!!!!!!!!!!!!!!!!!HAPPY VALENTINES DAY MY DEAR LOVE
-----------------------
Hey ! for your kind info My mobile will be switched off on Feb-14 to avoid unwanted proposal.So dont try to call on that day and intimate to Ur friends too.... Happy Valentines day !!
-----------------------
Wen i check my pocket i find sum coins, wen i check my purse i find sum rupes, but wen i check my heart i found"U"& realised HOW RICH I AM..HAPPY VELENTINE DAY
-----------------------
Its d month of Kisses,
surprises proposals n dates
chocolatees n gifts
hugs n Luvsongs,
Its FEB
Wishing u a Luv filled VALLENTINE month.
-----------------------
Breaking News:
My Heart Is For Sale
100% Discount For Your Girlfriends.
Last Date: 14 Feb Hurry!
Happy Valentine's day.
-----------------------
Increase Virtual Memory of Your Personal Computer - Xp Trics
When there is less physical memory than needed, then some virtual memory (virtual RAM)
is created on the disk. When there is a limit set to the size of
virtual memory usage and there is not enough of it present there is a
warning that frequently comes “Virtual Memory too low”.
Solution 1 :
This problem occurs when physical RAM + Virtual RAM (usually created on hard disk) together are not sufficient to take care of the currently needed RAM size, or this situation is about to arise. This usually happens when some fixed upper limit for Virtual Memory has been set. To solve this problem, you can either upgrade you physical RAM on your computer (recommended), or you can set the Virtual Memory to system managed size or you can do both. Setting Virtual Memory to system managed size means windows will set it to higher size whenever needed automatically,for this,
Right click on My computer --> goto properties, goto advanced tab, click on performance settings, now click on advanced, now click on advanced, now click on change button for Virtual memory.
Solution 1 :
This problem occurs when physical RAM + Virtual RAM (usually created on hard disk) together are not sufficient to take care of the currently needed RAM size, or this situation is about to arise. This usually happens when some fixed upper limit for Virtual Memory has been set. To solve this problem, you can either upgrade you physical RAM on your computer (recommended), or you can set the Virtual Memory to system managed size or you can do both. Setting Virtual Memory to system managed size means windows will set it to higher size whenever needed automatically,for this,
Right click on My computer --> goto properties, goto advanced tab, click on performance settings, now click on advanced, now click on advanced, now click on change button for Virtual memory.
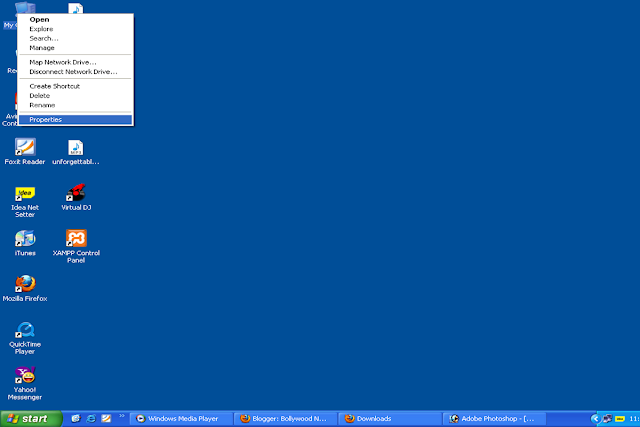
On this screen select the System managed size, press OK. After this re-start your computer. This will solve the virtual memory too low problem.
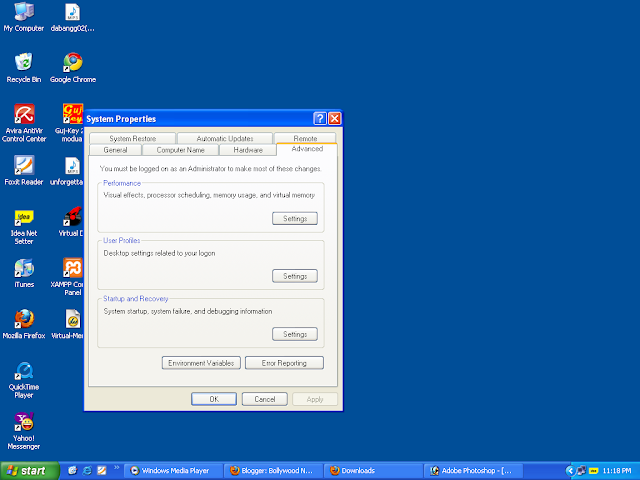
Solution 2: Recommended solution is to upgrade your physical memory (RAM) according to requirements of the kind of applications that you run on your computer. If you upgrade RAM or not, the following procedure will help prevent this warning by increasing the virtual memory limit.
- Right click on My computer icon and click properties.
- This will show system properties window. Click on a advanced tab and click on the settings button under heading performance.
- This will open performance options window. Click on advanced tab and click on change button under heading virtual memory.
- Check if the space available is set to some custom value or double the RAM size ?
- If it is not double the RAM memory size , then select the size option set the virtual memory size double the RAM Size and press set button and press OK button.
- Restart the computer.